Add all Contacts belonging to the Account
This article explains how to add a special option in the Contacts list lookup. When selected, this option will add all Contacts belonging to the Account.
Prerequisites
Implement a form customizer class and make sure GoMeddo is configured to use it, as described in the first two sections of the Quick Start Guide.
Make sure that the Account (B25__Account__c) field is visible on the reservation form, as described in Add custom fields to the Reservation form.
Make sure that the Reservation Contacts (B25__ReservationContact__c) related list is visible on the reservation form, as described in Displaying Dimension Junctions on the Reservation Form.
Search Handler
Implement the following search handler:
global with sharing class ContactSearchHandler extends B25.SearchHandler {
global override B25.SearchResultCollection getSearchResults(B25.SearchContext context) {
B25.SearchResultCollection resultCollection = new B25.SearchResultCollection();
if (context.getForm().getReservation().B25__Account__c != null) {
// Add a special search result
resultCollection.addSearchResult(
new B25.SearchResult('all-contacts', 'Add all Contacts of the current Account')
.setPreventDefault(true) // this line prevents GoMeddo from trying to add a record to the list
.setIcon('standard:contact_list')
);
}
// Now also include the default GoMeddo search results
resultCollection.addSearchResults(context.getDefaultResults().getSearchResults());
return resultCollection;
}
}
This handler first checks if the Account field on the reservation is set. If the Account is set, it adds a special option in the results that the user can select. Note the special option has an id of ‘all-contacts’. We will use this id later in the other handler to detect if the special option was selected.
Event Handler
Implement the following event handler:
global with sharing class ReservationContactHandler extends B25.FormEventHandler {
global override void handleEvent(B25.FormEvent event, B25.Form form) {
// Check if the Account has been set and selected option was the special option 'all-contacts'
B25__Reservation__c reservation = form.getReservation();
if (reservation.B25__Account__c == null || event.getNewValue() != 'all-contacts') {
return;
}
// Now add all Contacts linked to the Account
for (Contact contact : [SELECT Id FROM Contact WHERE AccountId = :reservation.B25__Account__c]) {
form.getRelatedList(B25__ReservationContact__c.SObjectType).addRecord(new B25__ReservationContact__c(
B25__Contact_Lookup__c = contact.Id
));
}
}
}
Customizer
Now add the following line to the customize method inside your customizer class:
form.getRelatedList(B25__ReservationContact__c.SObjectType).onSearch(new ContactSearchHandler());
form.getRelatedList(B25__ReservationContact__c.SObjectType).onAdd(new ReservationContactHandler());
This adds both your handlers to the Reservation Contact list lookup.
Test your solution
Go to the calendar.
Create a new reservation.
Select an account. Make sure this is an account with multiple contacts.
Place your cursor in the Contacts list lookup.
Select the special option 'Add all Contacts of the current Account'.
Notice that all contacts have been added.
Example of the final result
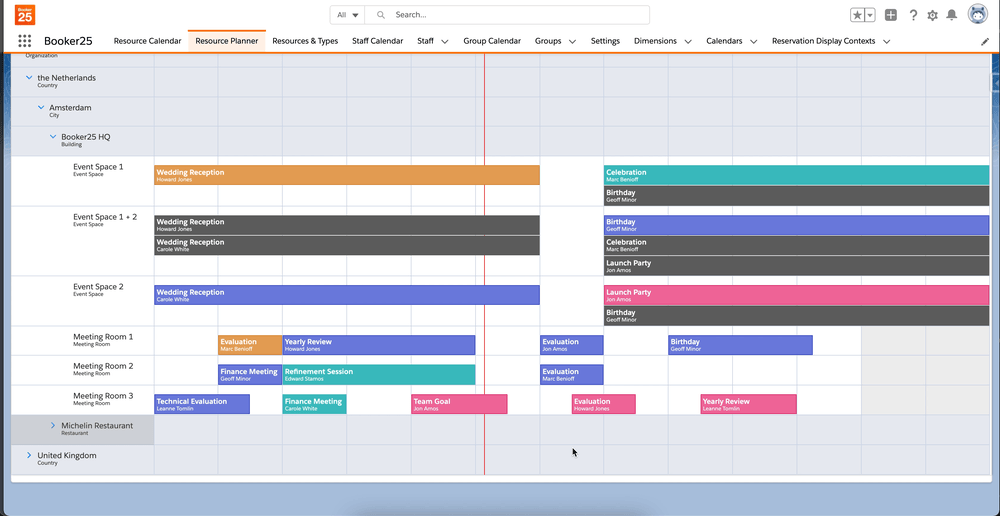