Apply custom price calculations
Use the following procedure to define custom price calculations on resources and services.
By default, GoMeddo executes the Default Price Calculation. You can overwrite this behavior by implementing your own price calculation. This article explains how to do that.
1. Create your custom price calculator class
Create a class that implements the interface B25.Util_PluginManager.ReservationPrice. Implementing that interface means the class must have a method with the following signature:
void calculate(B25.Util_PluginManager.ReservationPriceData)
This method will be called whenever a reservation is saved, or whenever the reservation form gets re-rendered. You can control when the reservation form gets re-rendered, more on that in a bit. The B25.Util_PluginManager.ReservationPriceData object that gets passed along to the calculate method has two properties that will help you to make your own price calculation.
Name | Type | Purpose |
---|---|---|
reservation | B25__Reservation__c | Contains the reservation as it is at the moment the calculate method is called. It will have all the fields populated that are visible on the reservation form. |
serviceCosts | Decimal | Contains the service costs as shown on the reservation form when you have the setting 'Services have a price' enabled. |
In the calculate method you will be able to set any fields on the reservation property record, such as the B25__Subtotal__c field, in order to control the final price of the reservation, or to start an approval process.
For the official documentation on how to write classes and how to implement interfaces go to: https://developer.salesforce.com/docs/atlas.en-us.apexcode.meta/apexcode/apex_classes.htm
Make sure your class is global, so that GoMeddo can instantiate it. See the code sample below.
2. Activate the custom price calculator class
Now, let GoMeddo know that it should use your custom class instead of the default price calculation
To do this:
Go to Setup
Search for Custom Settings
Click Manage next to System Setting
Click New
Name the record Reservation Price Calculator Class, and for String Value enter the name of your custom price calculator class
As long as this setting exists, GoMeddo will execute the calculate method every time the reservation form gets rerendered or when the reservation is saved.
3. (Optional) Control which fields trigger the calculation
By default, the following Reservation fields trigger the calculation whenever their value changes: Start, End, Base Price, Calculation Method, and Quantity. If you want to change which fields trigger the calculation, create a class that implements the interface B25.Util_PluginManager.ReservationPriceFields. Implementing that interface means the class must have a method with the following signature:
Set<String> getDependantFieldNames(B25.Util_PluginManager.ReservationPriceData)
To let GoMeddo know about the existence of the class, either place it in the same class as your custom price calculator class or create a new custom setting just as in step 2, except this time name the new record Reservation Price Fields Class. For String Value enter the name of the class you created.
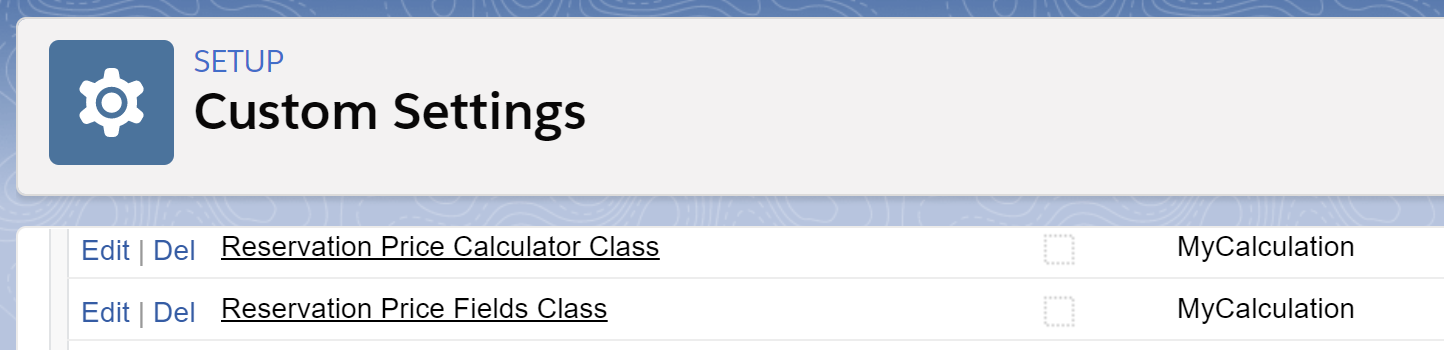
If you are using the external GoMeddo Widget to trigger custom price calculations, only the default fields will be passed.
Sample Code
The sample code below adds the account field as one of the fields triggering calculation, besides all the default fields.
Then, in the actual calculation method, it sets the price to zero if the linked account is an Installation Partner.
global class MyCalculation implements B25.Util_PluginManager.ReservationPrice, B25.Util_PluginManager.ReservationPriceFields {
public Set<String> getDependantFieldNames(B25.Util_PluginManager.ReservationPriceData data) {
return new Set<String>{
'B25__Account__c',
'B25__StartLocal__c',
'B25__EndLocal__c',
'B25__Base_Price__c',
'B25__Quantity__c',
'B25__Calculation_Method__c'
};
}
public void calculate(B25.Util_PluginManager.ReservationPriceData data) {
if (data.reservation.B25__Account__c == null) {
return;
}
Account linkedAccount = [
SELECT Type
FROM Account
WHERE Id = :data.reservation.B25__Account__c
];
if (linkedAccount.Type == 'Installation Partner') {
data.reservation.B25__Subtotal__c = 0;
}
}
}
Test code for class above
@IsTest
private class Test_MyCalculation {
@IsTest static void priceCalculation() {
Account testAccount = new Account(
Name = 'name',
Type = 'Installation Partner'
);
Database.insert(testAccount);
B25__Reservation__c testReservation = new B25__Reservation__C(
B25__Account__c = testAccount.Id,
B25__StartLocal__c = DateTime.newInstance(2017, 1, 1, 4, 0, 0),
B25__EndLocal__c = DateTime.newInstance(2017, 1, 2, 4, 0, 0),
B25__Subtotal__c = 100
);
Database.insert(testReservation);
B25.Util_PluginManager.ReservationPriceData rpd = new B25.Util_PluginManager.ReservationPriceData();
rpd.reservation = testReservation;
MyCalculation myCalculationClass = new MyCalculation();
//Test the DependantFieldName class and checks that there are 6 fields returned
Set<String> fields = myCalculationClass.getDependantFieldNames(rpd);
System.assertEquals(6, fields.size());
//Do the calculation and test that the price is 0 for this Account
myCalculationClass.calculate(rpd);
System.assertEquals(0, testReservation.B25__Subtotal__c);
}
}
Related articles
Related issues |
---|