Dynamic resource titles
We have covered setting up simple Resource Titles to display fields of your resource records (or other dimensions) on the calendar. It is also possible to calculate dynamic values on the fly, using your own Apex logic.
For configuring simple Resource Titles, see: Show fields on calendar resources
One example use case for dynamic titles is summing values of reservations visible in the current view, as shown in the screenshot below:
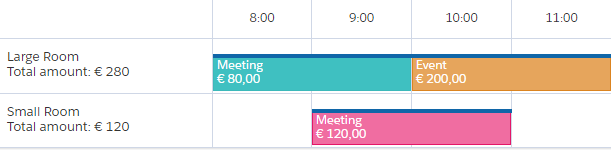
Only use dynamic titles on the multi resource calendar when there is no other way to achieve the same functionality (by using a formula field for example).
The multi resource calendar could potentially display a large number of resources, and dynamically recalculating the values every time the users view changes can negatively impact the user experience. This is of less concern on the single resource calendar but other options are almost always faster.
Create an Apex class
Write a global apex class that implements the B25.Util_PluginManager.DynamicResourceTitles interface.
Add the following method: Map<Id, String> calculateResourceTitles(B25.Util_PluginManager.DynamicResourceTitleData dataObj);
See the Interface Reference section below for more details.Save the class.
Create a Resource Title record
Activate your implementation on a calendar by creating a Resource Title record for it.
Go to the Calendars tab and find the calendar that you want to apply the dynamic titles on.
On the Related subtab, find the Resource Titles related list and click New.
Make sure to select Resource Title when prompted for the record type.
For the Title Name, enter the name of the class you created in the previous section.
Check the Dynamic checkbox.
Interface Reference
The DynamicResourceTitles interface enforces one method: calculateResourceTitles. This method takes a B25.Util_PluginManager.DynamicResourceTitleData object as input. This object contains the following information:
Property | Type | Description |
---|---|---|
viewName | String | The name of the current view that the user sees on the calendar. |
startDatetime | Datetime | The start of the current view that the user sees on the calendar. |
endDatetime | Datetime | The end of the current view that the user sees on the calendar. |
resourceToVisibleReservations | Map<Id, List<Id>> | Map of resource ids to a list of the ids of the reservations that are currently visible to the user. This list will also contain any unshared reservations that are visible to the user (if "Show greyed out blocks on the calendar for unshared reservations" is enabled). If you want to query the unshared reservations as well as the shared ones the implementation class needs to be without sharing. |
resourceToHiddenReservations | Map<Id, List<Id>> | Map of resource ids to a list of the ids of the reservations that are currently hidden from the user by reservation filters on the calendar. |
Using this information the method should calculate the values for each resource and return a Map<Id, String> containing a mapping of the resource to the value that should be displayed on the calendar.
The following example displays the number of currently visible reservations in each resource.
Example
global with sharing class DynamicTitleImplementation implements B25.Util_PluginManager.DynamicResourceTitles {
global Map<Id, String> calculateResourceTitles(B25.Util_PluginManager.DynamicResourceTitleData dataObj) {
// all the reservations that are currently visible to the user, mapped to their resource
Map<Id, List<Id>> visibleReservations = dataObj.resourceToVisibleReservations;
// the values we are going to return, mapped by resource
Map<Id, String> output = new Map<Id, String>();
// loop over each resource
for (Id resourceId : visibleReservations.keySet()) {
// get the visible reservations in the current resource
List<Id> reservationIds = visibleReservations.get(resourceId);
// how many are there?
Integer numberOfReservations = reservationIds.size();
// add the result to the output map
output.put(resourceId, String.valueOf(numberOfReservations));
}
return output;
}
}
Unit testing dynamic resource titles
To unit test your implementation you can construct a B25.Util_PluginManager.DynamicResourceTitleData set the parameters required by your implementation and call your calculateResourceTitles implementation.
Example
@IsTest
public with sharing class TestDynamic {
@IsTest
public static void calculateResourceTitles() {
// Create a new data object and set the data required by your implemetation
String fakeId = '000000000000000000';
B25.Util_PluginManager.DynamicResourceTitleData data = new B25.Util_PluginManager.DynamicResourceTitleData();
data.resourceToVisibleReservations = new Map<Id, List<Id>>();
data.resourceToVisibleReservations.put('000000000000000001', new List<Id>{fakeId, fakeId, fakeId});
data.resourceToVisibleReservations.put('000000000000000002', new List<Id>{fakeId, fakeId});
// Call the implementation
DynamicTitleImplementation implementation = new DynamicTitleImplementation();
Map<Id, String> result = implementation.calculateResourceTitles(data);
System.assertEquals('3', result.get('000000000000000001'));
System.assertEquals('2', result.get('000000000000000002'));
}
}
Related articles
Related issues |
---|